ConsentiumNow Library: Seamless ESP-NOW Communication for IoT Devices
The ConsentiumNow library provides seamless ESP-NOW communication between ESP32-compatible devices. It is a powerful tool designed to…
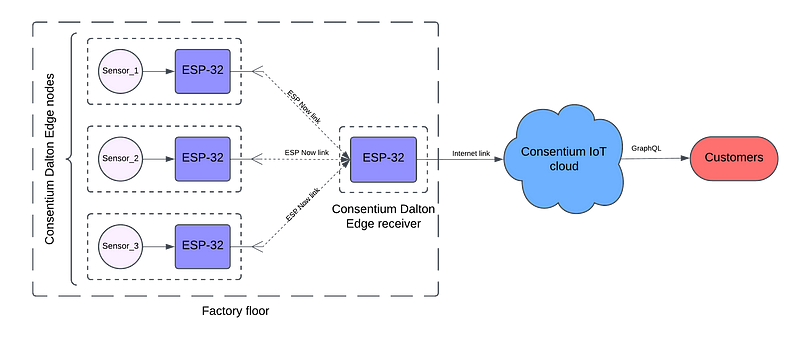
The ConsentiumNow library provides seamless ESP-NOW communication between ESP32-compatible devices. It is a powerful tool designed to facilitate real-time, reliable data exchange for IoT applications using custom user-defined data structures. Built with simplicity and flexibility in mind, the library is lightweight yet robust, supporting a wide range of IoT use cases.
Key Features
- Lightweight and flexible ESP-NOW integration.
- Customizable data structures for specific applications.
- Supports one-to-one and one-to-many communication.
- Easy-to-use API for sending and receiving data.
- Highly efficient for low-latency IoT communication.
Getting Started
Prerequisites
Before you begin, ensure you have the following:
- Hardware: ESP32-compatible boards (minimum two for testing).
- Software: Arduino IDE configured for ESP32 development.
- Basic Knowledge: Familiarity with the ESP-NOW protocol and Arduino programming basics.
Installation
- Clone this repository or download it as a ZIP.
- Open the Arduino IDE and navigate to
Sketch > Include Library > Add .ZIP Library
. - Select the ConsentiumNow library ZIP file.
Alternatively, search for ConsentiumNow in Arduino libraries.
Usage Examples
1. Basic Sender
#include <ConsentiumNow.h>
// Define the data structure
struct SensorData {
float temperature; // Temperature in °C
float humidity; // Humidity in %
};
// Create an instance of ConsentiumNow for sending
ConsentiumNow<SensorData> consentiumSender;
// Replace with the receiver's MAC address
uint8_t receiverMac[] = {0x7C, 0x9E, 0xBD, 0x66, 0x7A, 0x0C};
void setup() {
Serial.begin(115200);
consentiumSender.sendBegin(receiverMac); // Initialize sender
Serial.println("Sender initialized.");
}
void loop() {
SensorData data;
data.temperature = random(200, 300) / 10.0; // Random temperature (20.0 - 30.0)
data.humidity = random(400, 600) / 10.0; // Random humidity (40.0 - 60.0)
consentiumSender.sendData(data);
Serial.printf("Sent Data - Temperature: %.2f, Humidity: %.2f\n",
data.temperature, data.humidity);
delay(2000); // Send every 2 seconds
}
2. Basic Receiver
#include <ConsentiumNow.h>
// Define the data structure
struct SensorData {
float temperature; // Temperature in °C
float humidity; // Humidity in %
};
// Create an instance of ConsentiumNow for receiving
ConsentiumNow<SensorData> consentiumReceiver;
void setup() {
Serial.begin(115200);
consentiumReceiver.receiveBegin(); // Initialize receiver
Serial.println("Receiver ready to receive data.");
}
void loop() {
if (consentiumReceiver.isDataAvailable()) {
SensorData data = consentiumReceiver.getReceivedData();
Serial.printf("Received Data - Temperature: %.2f, Humidity: %.2f\n",
data.temperature, data.humidity);
}
delay(500); // Check every 500ms
}
Multi-Sender to One Receiver
- Configure the receiver to recognize multiple senders.
- Use
addPeer()
to add the MAC addresses of each sender
void setup() {
consentiumReceiver.receiveBegin();
uint8_t sender1Mac[] = {0x7C, 0x9E, 0xBD, 0x66, 0x7A, 0x0C};
uint8_t sender2Mac[] = {0x24, 0x6F, 0x28, 0x18, 0x2B, 0x4A};
consentiumReceiver.addPeer(sender1Mac);
consentiumReceiver.addPeer(sender2Mac);
Serial.println("Ready to receive data from multiple senders.");
}
Custom Data Structures
Modify the struct
to include additional fields based on your use case.
struct ExtendedSensorData {
float temperature;
float humidity;
int deviceID; // Device identifier
long timestamp; // Timestamp in ms
};
Use the custom structure in both sender and receiver implementations.
FAQs
1. How many devices can communicate simultaneously using ESP-NOW?
ESP-NOW supports up to 10 peers (devices) in station mode.
2. Can I encrypt the data sent using this library?
Currently, the library does not support encryption. Use HTTPS or secure layers for sensitive data.
3. How reliable is ESP-NOW for IoT applications?
ESP-NOW is highly reliable for short-range, low-latency communication, making it ideal for IoT.