Building an IoT-Based Sine Wave Predictor with EdgeNeuron — Consentium TinyML Library
In this comprehensive guide, we will demonstrate how to use the Consentium TinyML library, specifically the EdgeNeuron framework, with…
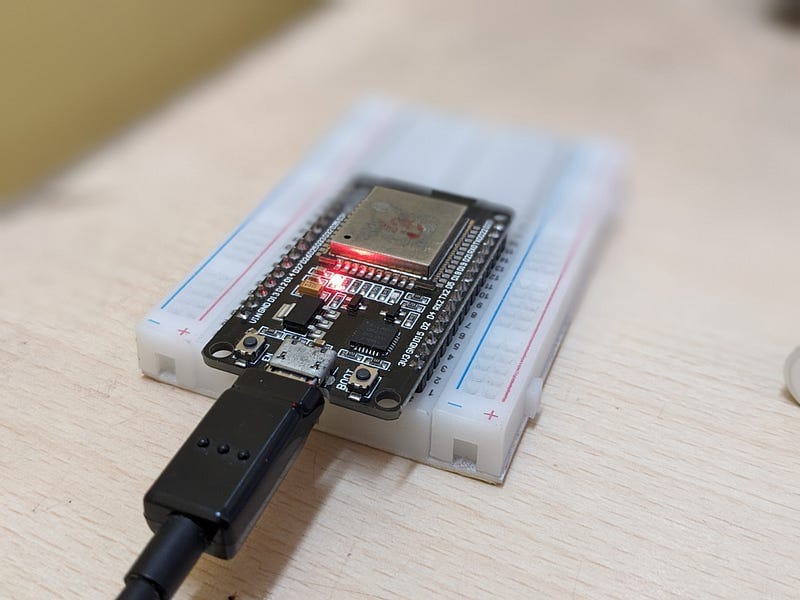
In this comprehensive guide, we will demonstrate how to use the Consentium TinyML library, specifically the EdgeNeuron framework, with microcontroller platforms like ESP32 or Raspberry Pi Pico W. This example focuses on deploying a TensorFlow Lite model to predict sine wave values on a microcontroller while seamlessly integrating with IoT boards for real-time data transmission.
Overview
The tutorial will walk you through:
- Building the model and exporting it using edgemodelkit [Google colab]
- Setting up WiFi connectivity and transmitting IoT data using EdgeNeuron.
- Initializing and running a TensorFlow Lite model for sine wave prediction.
- Transmitting predicted data to the Consentium IoT cloud for analysis and monitoring.
Key Components
Libraries Used
- EdgeNeuron.h: The core library that provides streamlined TinyML functionalities to work with TensorFlow Lite on microcontrollers. It handles model initialization, inference, and memory management.
- ConsentiumThings.h: Manages IoT operations like WiFi initialization, data formatting, and transmission to Consentium IoT cloud servers.
Core Variables
IoT Object Initialization
ConsentiumThingsDalton board;
This object is responsible for handling WiFi connectivity and data transmission.
WiFi Credentials
const char* ssid = "Your_SSID";
const char* pass = "Your_PASSWORD";
These variables store your network credentials for connectivity.
API Keys
const char* SendApiKey = "Your_Send_API_Key";
const char* BoardApiKey = "Your_Board_API_Key";
These keys authenticate and identify your board when transmitting data to the Consentium IoT cloud.
TensorFlow Lite Variables
uint8_t tensor_arena[kTensorArenaSize];
const int kTensorArenaSize = 2048;
The tensor arena is the memory buffer where the TensorFlow Lite model operates. Ensure this is large enough for your model.
Code Breakdown
1. Setup Function
This function initializes WiFi connectivity and the TensorFlow Lite model using EdgeNeuron.
void setup() {
board.initWiFi(ssid, pass); // Initialize WiFi
board.beginSend(SendApiKey, BoardApiKey); // Start data transmission
// Initialize the model
if (!initializeModel(model, tensor_arena, kTensorArenaSize)) {
while (true); // Halt if model initialization fails
}
}
board.initWiFi(ssid, pass);
: Establishes connection to the WiFi network.board.beginSend(SendApiKey, BoardApiKey);
: Authenticates the board for secure data transmission.initializeModel(...)
: Prepares the TensorFlow Lite model for execution using EdgeNeuron’s efficient memory handling.
2. Main Loop
This loop continuously predicts the sine of x
and sends the prediction along with the actual value to the server for comparison.
void loop() {
if (x > 6.28) {
x = 0.0;
}
setModelInput(x, 0); // Set model input for inference
if (!runModelInference()) {
return; // If inference fails, exit
}float y_predicted = getModelOutput(0); // Model’s prediction
float y_actual = sin(x); // Actual sine value
vector<double> sensorValues = {x, y_predicted, y_actual};
const char* sensorInfo[] = {"Input", "Predicted", "Actual"};board.sendData(sensorValues, sensorInfo, LOW_PRE); // Transmit datax += step; // Increment x
delay(interval); // Wait before the next prediction
}
setModelInput(x, 0);
: Sets the input for the TensorFlow Lite model.runModelInference();
: Runs the inference process using EdgeNeuron’s optimized inference engine.getModelOutput(0);
: Retrieves the predicted value from the model.board.sendData(...);
: Sends data to the Consentium IoT cloud in a lightweight format.
Additional Notes
WiFi Configuration
Ensure your SSID and password are correctly set. The initWiFi()
function establishes a connection to the network before any model inference.
API Keys
The SendApiKey
and BoardApiKey
must be valid and correctly configured to allow data transmission to the Consentium IoT cloud.
TensorFlow Lite Model
Ensure the model (model.h
) is compiled for microcontroller usage and fits within the tensor_arena
. Use tools like xxd
to convert the model to a C array.
Potential Modifications
- Step Size Adjustment: Modify the
x
increment step size for more granular or coarser predictions. - Delay Configuration: Adjust the
delay(interval);
to control the frequency of data transmission. - Memory Optimization: Increase
kTensorArenaSize
or reduce model complexity if you encounter memory issues.
Example Use Case
This example demonstrates how to predict sine wave values between 0
and 2π
using EdgeNeuron, the TinyML framework from Consentium IoT. Such a framework is ideal for developing low-power, high-efficiency IoT applications where real-time data analysis is critical.
License
This project is licensed under the MIT License. Redistribution or modification of this code must include the above information from Consentium IoT and EdgeNeuron.